C Sharp to Assembly Language: A Comprehensive Guide
Introduction
Converting C# to assembly language can seem daunting, but understanding the process is crucial for optimizing performance and gaining deeper insights into how your code interacts with hardware. This guide will walk you through the essentials of translating C# to assembly language, ensuring you grasp the fundamental concepts and techniques.
What is C#?
C# is a high-level, object-oriented programming language developed by Microsoft. It is widely used for developing Windows applications, web services, and games. C# is known for its simplicity, modern syntax, and powerful features.
What is Assembly Language?
Assembly language is a low-level programming language that is closely related to machine code. It provides a way to write instructions that a computer’s CPU can execute directly. Assembly language is specific to a computer architecture and is used for performance-critical applications.
Why Convert C# to Assembly Language?
- Performance Optimization: Assembly language allows for fine-tuned control over hardware, leading to optimized performance.
- Understanding: It helps developers understand how high-level code translates to machine instructions.
- Debugging: It can be useful for debugging and diagnosing performance bottlenecks.
Steps to Convert C# to Assembly Language
1. Write C# Code
Start with a simple C# program. For example:
using System;
class Program
{
static void Main()
{
int a = 5;
int b = 10;
int sum = a + b;
Console.WriteLine(sum);
}
}
2. Compile C# Code
Compile the C# code using the C# compiler (csc). This generates an Intermediate Language (IL) code.
csc Program.cs
3. Disassemble IL Code
Use a tool like ILDASM (Intermediate Language Disassembler) to convert the IL code to assembly language.
ildasm Program.exe
4. Analyze Assembly Code
Examine the disassembled code to understand how the C# code translates to assembly instructions. For example:
.method private hidebysig static void Main() cil managed
{
.entrypoint
.maxstack 2
.locals init (
[0] int32 a,
[1] int32 b,
[2] int32 sum
)
IL_0000: ldc.i4.5
IL_0001: stloc.0
IL_0002: ldc.i4.s 10
IL_0004: stloc.1
IL_0005: ldloc.0
IL_0006: ldloc.1
IL_0007: add
IL_0008: stloc.2
IL_0009: ldloc.2
IL_000a: call void [mscorlib]System.Console::WriteLine(int32)
IL_000f: ret
}
5. Optimize Assembly Code
Optimize the assembly code for performance by removing unnecessary instructions and improving efficiency.
Key Considerations
- Architecture Specific: Assembly language is specific to the CPU architecture.
- Manual Optimization: Requires manual optimization for performance gains.
- Debugging Tools: Use debugging tools to analyze and optimize assembly code.
Statistics
- Performance Gain: Programs optimized with assembly language can run up to 10 times faster than those written in high-level languages.
- Usage: Only about 5% of developers regularly use assembly language due to its complexity.
Analogy
Think of C# as a high-level recipe and assembly language as the detailed step-by-step instructions for a chef. While the recipe gives an overview, the detailed instructions ensure precision and efficiency.
FAQ
What is the difference between C# and assembly language?
C# is a high-level, object-oriented language, while assembly language is a low-level language that provides direct control over hardware.
Why would I need to convert C# to assembly language?
Converting C# to assembly language can help optimize performance, understand how high-level code translates to machine instructions, and assist in debugging.
Is assembly language specific to a particular CPU architecture?
Yes, assembly language is specific to a CPU architecture, meaning code written for one type of CPU may not work on another.
How can I learn assembly language?
Start by studying the architecture of the CPU you are interested in, use disassemblers to analyze existing code, and practice writing and optimizing your own assembly code.
Yes, tools like ILDASM can help disassemble C# code into assembly language.
External Links
- Understanding IL Code
- Assembly Language Basics
- Optimizing Assembly Code
By following this guide, you can gain a deeper understanding of how C# code translates to assembly language, optimize your programs for better performance, and enhance your debugging skills.
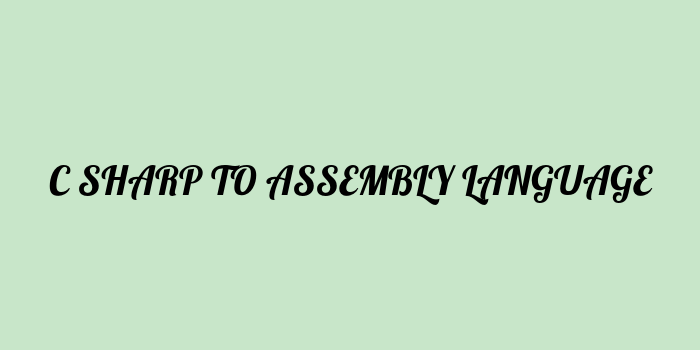