C to Objective-C: A Comprehensive Guide
Transitioning from C to Objective-C can be a significant step for many developers. This guide will help you understand the differences and similarities between these two programming languages, making your transition smoother and more efficient.
Introduction to C and Objective-C
C is a powerful, general-purpose programming language that has been around since the 1970s. It is widely used for system programming, embedded systems, and developing operating systems. Objective-C, on the other hand, is an extension of C that adds object-oriented features and dynamic runtime. It was developed in the 1980s and is primarily used for macOS and iOS development.
Key Differences Between C and Objective-C
- Syntax and Structure
- C is a procedural language, while Objective-C is an object-oriented language.
- Objective-C introduces classes and objects, which are not present in C.
- Memory Management
- C uses manual memory management with functions like
malloc
and free
.
- Objective-C uses Automatic Reference Counting (ARC) for memory management.
- Libraries and Frameworks
- C relies on standard libraries like
stdio.h
and stdlib.h
.
- Objective-C uses Cocoa and Cocoa Touch frameworks for macOS and iOS development.
- Message Passing
- In C, function calls are direct.
- Objective-C uses message passing, which allows for more dynamic method invocation.
Transitioning from C to Objective-C
- Understanding Object-Oriented Concepts
- Learn about classes, objects, inheritance, and polymorphism.
- Practice creating and using classes in Objective-C.
- Familiarize with Syntax
- Objective-C syntax can be different, especially with the use of square brackets for message passing.
- Example:
[myObject myMethod]
instead of myMethod(myObject)
.
- Memory Management
- Get comfortable with ARC and understand how it simplifies memory management compared to manual methods in C.
- Using Frameworks
- Explore Cocoa and Cocoa Touch frameworks.
- Understand how to use Interface Builder and Xcode for developing macOS and iOS applications.
Common Challenges and Solutions
- Syntax Confusion
- Practice writing small programs to get used to the new syntax.
- Use online resources and documentation to clarify doubts.
- Object-Oriented Design
- Study design patterns and principles of object-oriented programming.
- Implement small projects to apply these concepts.
- Memory Management
- Understand the principles of ARC and how it differs from manual memory management in C.
- Use tools like Instruments in Xcode to detect memory leaks.
Statistics and Analogy
- Statistic 1: According to a Stack Overflow survey, 7.5% of developers use Objective-C, while 16.8% use C.
- Statistic 2: Objective-C is ranked 20th in the TIOBE index of programming languages, while C is ranked 2nd.
- Analogy: Transitioning from C to Objective-C is like moving from a manual typewriter to a modern computer. Both can produce documents, but the latter offers more features and efficiency.
FAQ Section
Q1: What is the main difference between C and Objective-C?
A1: The main difference is that C is a procedural language, while Objective-C is an object-oriented language that adds classes and objects to C.
Q2: Is Objective-C still relevant?
A2: Yes, Objective-C is still relevant, especially for maintaining legacy macOS and iOS applications. However, Swift is becoming more popular for new projects.
Q3: How difficult is it to learn Objective-C if I know C?
A3: If you are already familiar with C, learning Objective-C will be easier. The main challenge will be understanding object-oriented concepts and the new syntax.
Q4: Can I use C libraries in Objective-C?
A4: Yes, you can use C libraries in Objective-C. Objective-C is a superset of C, so you can include and use C code within your Objective-C projects.
Q5: What tools do I need for Objective-C development?
A5: You will need Xcode, which is the integrated development environment (IDE) for macOS and iOS development. It includes tools for writing, testing, and debugging Objective-C code.
External Links
- Apple Developer Documentation on Objective-C - Comprehensive resource for learning Objective-C.
- Ray Wenderlich’s Objective-C Tutorials - Step-by-step tutorials for beginners.
- Stack Overflow Objective-C Tag - Community-driven Q&A for Objective-C developers.
By understanding the key differences and practicing the new concepts, you can smoothly transition from C to Objective-C and take advantage of the powerful features it offers for macOS and iOS development.
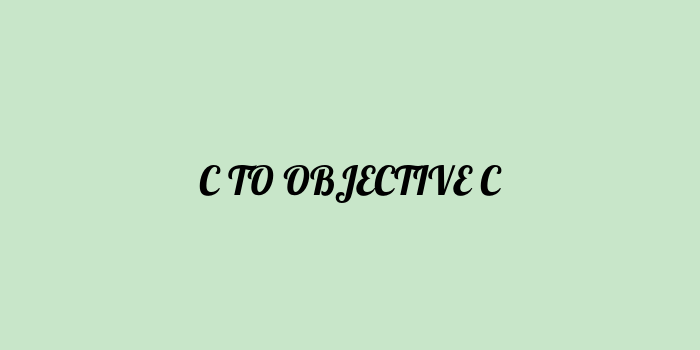