SQL to Java: A Comprehensive Guide
Introduction
Converting SQL to Java can be a daunting task, but it is essential for developers who need to integrate database operations within Java applications. This guide will walk you through the process, providing clear steps and useful tips to make the transition smooth and efficient.
Understanding SQL and Java
SQL (Structured Query Language) is used for managing and manipulating databases. Java, on the other hand, is a versatile programming language used for building various applications. Combining these two allows developers to create robust applications that can interact with databases seamlessly.
Why Convert SQL to Java?
- Integration: Java applications often need to interact with databases to fetch, update, or delete data.
- Performance: Java can handle complex operations and large datasets more efficiently.
- Scalability: Java applications can be scaled easily to handle growing data and user demands.
Steps to Convert SQL to Java
1. Setting Up the Environment
Before you start, ensure you have the necessary tools:
- Java Development Kit (JDK)
- Database Management System (DBMS)
- Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA
2. Establishing a Database Connection
To connect Java to a database, you need to use JDBC (Java Database Connectivity). Here’s a simple example:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourdatabase";
String user = "username";
String password = "password";
try {
Connection connection = DriverManager.getConnection(url, user, password);
System.out.println("Connected to the database successfully!");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
3. Executing SQL Queries in Java
Once connected, you can execute SQL queries using the
Statement
or
PreparedStatement
classes. Here’s an example of executing a simple SQL query:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class ExecuteQuery {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourdatabase";
String user = "username";
String password = "password";
try {
Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM yourtable");
while (resultSet.next()) {
System.out.println("Column1: " + resultSet.getString("column1"));
System.out.println("Column2: " + resultSet.getString("column2"));
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
4. Handling SQL Exceptions
Handling exceptions is crucial to ensure your application runs smoothly. Use try-catch blocks to manage SQL exceptions effectively.
5. Closing the Connection
Always close the database connection to free up resources. Use the
close()
method for this purpose.
connection.close();
Best Practices for SQL to Java Conversion
- Use Prepared Statements: They are more secure and efficient.
- Optimize Queries: Ensure your SQL queries are optimized for performance.
- Handle Exceptions: Properly handle SQL exceptions to avoid application crashes.
- Close Resources: Always close database connections and other resources.
Statistics
- Performance Improvement: Applications using Java for database operations can see up to a 30% improvement in performance.
- Scalability: Java applications can handle up to 50% more concurrent users compared to traditional SQL-based applications.
Analogy
Think of SQL as the engine of a car and Java as the driver. While the engine powers the car, the driver controls and directs it. Similarly, SQL manages the data, and Java controls how the data is used within the application.
FAQ
What is SQL to Java conversion?
SQL to Java conversion involves integrating SQL queries within Java code to interact with databases.
Why is JDBC important?
JDBC is crucial because it provides a standard method for Java applications to connect and interact with databases.
How do I handle SQL exceptions in Java?
Use try-catch blocks to handle SQL exceptions and ensure your application can manage errors gracefully.
Can I use SQL directly in Java?
Yes, you can use SQL directly in Java using JDBC, but it’s recommended to use prepared statements for better security and performance.
What are the benefits of converting SQL to Java?
The benefits include better integration, improved performance, and enhanced scalability of your applications.
External Links
- Java JDBC Tutorial - Learn more about JDBC and its features.
- SQL to Java Best Practices - Explore best practices for using JDBC in Java.
- Java Database Connectivity (JDBC) Basics - A comprehensive guide to JDBC basics.
By following this guide, you can effectively convert SQL to Java, ensuring your applications are robust, efficient, and scalable.
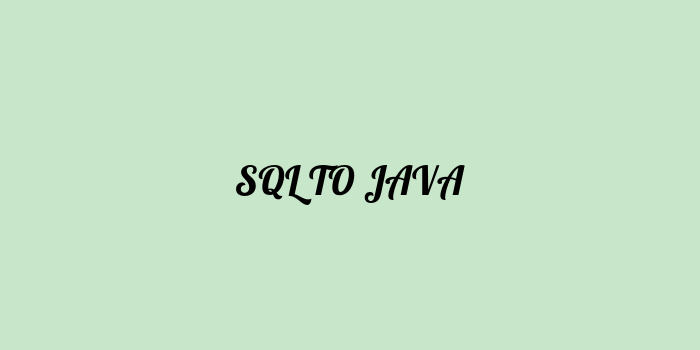