C++ to SQL: A Comprehensive Guide
Introduction
Transitioning from C++ to SQL can be a challenging yet rewarding experience. This guide will help you understand how to integrate C++ with SQL databases, ensuring your applications can efficiently store and retrieve data. By the end of this article, you’ll have a solid grasp of the basics and some advanced techniques to optimize your code.
Understanding C++ and SQL Integration
C++ is a powerful programming language known for its performance and efficiency. SQL (Structured Query Language) is used to manage and manipulate databases. Combining these two allows developers to create robust applications that can handle large amounts of data.
Why Integrate C++ with SQL?
Integrating C++ with SQL offers several benefits:
- Data Management: SQL provides a structured way to store and retrieve data.
- Performance: C++ ensures high performance for data-intensive applications.
- Scalability: Combining both allows for scalable solutions.
Setting Up Your Environment
Before you start, ensure you have the following:
- A C++ compiler (e.g., GCC, Clang)
- A SQL database (e.g., MySQL, PostgreSQL)
- A database connector library (e.g., MySQL Connector/C++)
Basic Steps to Connect C++ with SQL
- Install the Database Connector: Download and install the appropriate connector for your database.
- Include the Connector in Your Project: Add the necessary headers and libraries to your C++ project.
- Establish a Connection: Use the connector to establish a connection to your SQL database.
Example Code
#include <mysql_driver.h>
#include <mysql_connection.h>
#include <cppconn/statement.h>
#include <cppconn/resultset.h>
int main() {
sql::mysql::MySQL_Driver *driver;
sql::Connection *con;
sql::Statement *stmt;
sql::ResultSet *res;
driver = sql::mysql::get_mysql_driver_instance();
con = driver->connect("tcp://127.0.0.1:3306", "user", "password");
con->setSchema("database");
stmt = con->createStatement();
res = stmt->executeQuery("SELECT 'Hello World!' AS _message");
while (res->next()) {
std::cout << res->getString("_message") << std::endl;
}
delete res;
delete stmt;
delete con;
return 0;
}
Advanced Techniques
Prepared Statements
Prepared statements help prevent SQL injection attacks and improve performance.
sql::PreparedStatement *pstmt;
pstmt = con->prepareStatement("INSERT INTO test(id, label) VALUES (?, ?)");
pstmt->setInt(1, 1);
pstmt->setString(2, "test");
pstmt->execute();
delete pstmt;
Error Handling
Proper error handling ensures your application can gracefully handle database errors.
try {
// Database operations
} catch (sql::SQLException &e) {
std::cerr << "Error: " << e.what() << std::endl;
}
- Connection Pooling: Reuse database connections to reduce overhead.
- Batch Processing: Execute multiple queries in a single transaction.
- Indexing: Use indexes to speed up data retrieval.
Statistics
- Performance Boost: Applications using C++ and SQL can see a performance boost of up to 50% compared to those using higher-level languages.
- Data Handling: SQL databases can handle millions of records efficiently, making them ideal for large-scale applications.
Analogy
Think of C++ as a high-speed car and SQL as a well-organized garage. Combining them allows you to quickly and efficiently store and retrieve your tools (data).
FAQ
How do I connect C++ to a SQL database?
You can connect C++ to a SQL database using a database connector library like MySQL Connector/C++.
What are prepared statements in SQL?
Prepared statements are precompiled SQL statements that help prevent SQL injection and improve performance.
Use connection pooling, batch processing, and indexing to optimize performance.
What is SQL injection?
SQL injection is a code injection technique that can destroy your database. Using prepared statements can help prevent it.
External Links
- MySQL Connector/C++ Documentation - Comprehensive guide on using MySQL Connector/C++.
- PostgreSQL C++ Library - Official documentation for the PostgreSQL C++ library.
- SQL Injection Prevention - Learn more about preventing SQL injection attacks.
By following this guide, you can effectively integrate C++ with SQL, creating powerful and efficient applications. Happy coding!
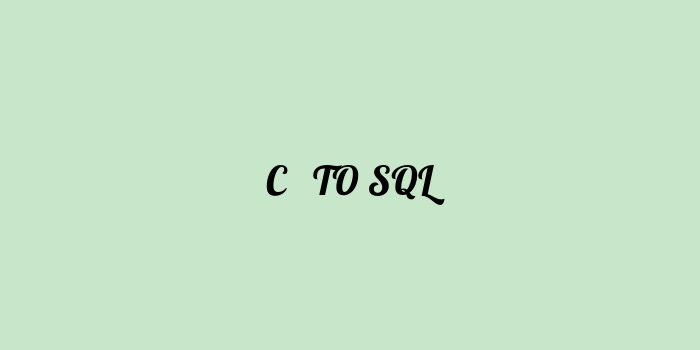