Python to SQL: A Comprehensive Guide
Introduction
Python and SQL are two of the most popular programming languages used in data science and web development. Python is known for its simplicity and versatility, while SQL is the standard language for managing and querying databases. This article will guide you through the process of converting Python code to SQL queries, ensuring you can efficiently manage your data.
Why Convert Python to SQL?
Python is excellent for data manipulation and analysis, but when it comes to querying large datasets, SQL is more efficient. Converting Python to SQL can help you leverage the strengths of both languages, making your data operations faster and more efficient.
How to Convert Python to SQL
1. Using SQLAlchemy
SQLAlchemy is a powerful library that allows you to interact with databases using Python. It provides a high-level ORM (Object-Relational Mapping) and a low-level SQL expression language.
from sqlalchemy import create_engine, MetaData, Table
engine = create_engine('sqlite:///example.db')
metadata = MetaData()
users = Table('users', metadata, autoload=True, autoload_with=engine)
2. Using Pandas
Pandas is a popular data manipulation library in Python. You can use it to read SQL queries into DataFrames and vice versa.
import pandas as pd
from sqlalchemy import create_engine
engine = create_engine('sqlite:///example.db')
df = pd.read_sql('SELECT * FROM users', engine)
3. Using SQLite3
SQLite3 is a lightweight database that comes with Python. It’s perfect for small to medium-sized applications.
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
Best Practices for Converting Python to SQL
1. Optimize Queries
Ensure your SQL queries are optimized for performance. Use indexes and avoid unnecessary columns.
2. Use Parameterized Queries
Always use parameterized queries to prevent SQL injection attacks.
cursor.execute('SELECT * FROM users WHERE id=?', (user_id,))
3. Handle Exceptions
Always handle exceptions to ensure your application can gracefully recover from errors.
try:
cursor.execute('SELECT * FROM users')
except sqlite3.Error as e:
print(f"An error occurred: {e}")
Statistics
- According to a survey by Stack Overflow, SQL is the third most popular language among developers.
- A study by IBM found that data scientists spend 80% of their time cleaning and preparing data, tasks that can be streamlined by using SQL.
Analogy
Think of Python as a Swiss Army knife and SQL as a scalpel. Python is versatile and can handle many tasks, but when you need precision and efficiency in data querying, SQL is the tool to use.
FAQ
What is the difference between Python and SQL?
Python is a general-purpose programming language, while SQL is specifically designed for managing and querying databases.
Can I use Python and SQL together?
Yes, you can use libraries like SQLAlchemy and Pandas to integrate Python with SQL databases.
Is SQL faster than Python for data queries?
Yes, SQL is generally faster for querying large datasets because it is optimized for such operations.
How do I prevent SQL injection in Python?
Use parameterized queries to prevent SQL injection attacks.
What are some common libraries for using SQL in Python?
SQLAlchemy, Pandas, and SQLite3 are some of the most commonly used libraries.
External Links
- SQLAlchemy Documentation
- Pandas Documentation
- SQLite3 Documentation
By following this guide, you can effectively convert Python code to SQL queries, optimizing your data operations and making your applications more efficient.
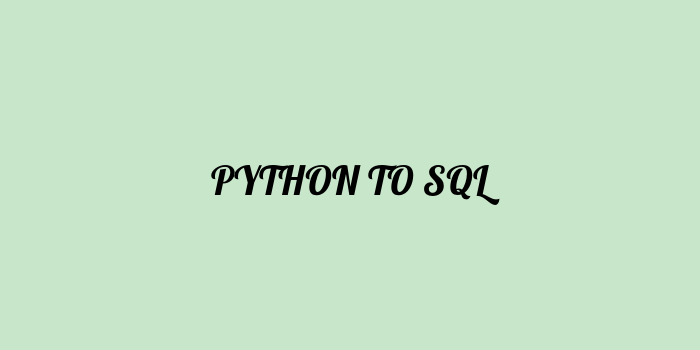