Effortlessly Convert Java to NoSQL - Try Our Tool Now!
Effortlessly convert Java objects to NoSQL databases with our powerful tool. Simplify data migration and boost efficiency. Try it today for seamless integration!
Source Code
Converted Code
Output will appear here...
Transform your Java applications with our Java to NoSQL tool, designed to seamlessly integrate NoSQL databases into your projects for enhanced scalability and performance. Perfect for developers seeking flexible data models and real-time analytics, this tool simplifies the transition from traditional databases to modern NoSQL solutions. Boost your applications efficiency and future-proof your architecture today.
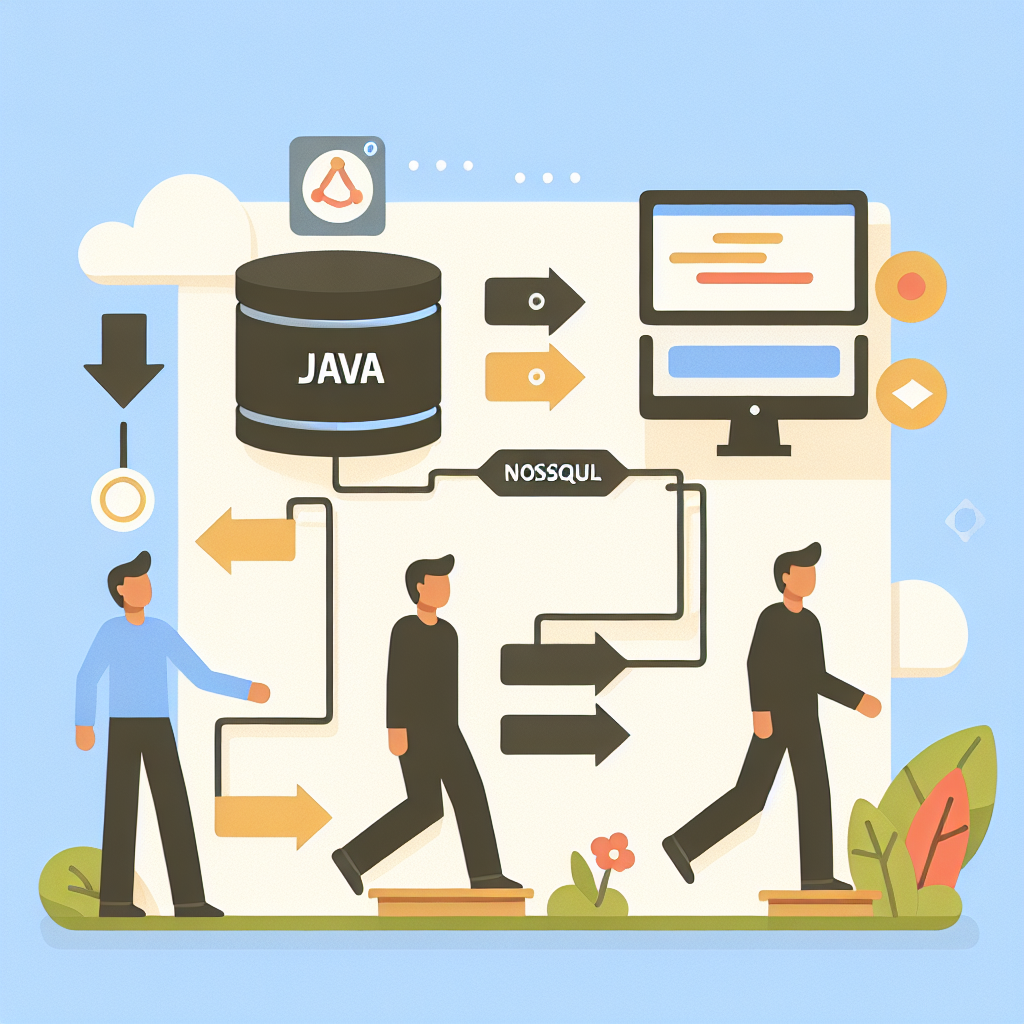
Java to NoSQL: Seamlessly Transition Your Data Management Link to this section #
Leveraging the right tools to convert Java applications to use NoSQL databases can enhance performance and scalability. Our 'Java to NoSQL' tool offers a streamlined approach to transition your data models, ensuring efficient and optimized database management.
Key Features Link to this section #
- Data Model Conversion: Automatically transform Java objects to NoSQL-compatible formats like JSON or BSON.
- Schema Evolution: Adapt to NoSQL's flexible schema, allowing dynamic data structures without predefined models.
- Query Translation: Convert SQL queries to NoSQL syntax, supporting popular databases like MongoDB, Cassandra, and Couchbase.
Why Use This Tool? Link to this section #
- Simplified Migration: Minimize manual coding efforts with automated data mapping.
- Enhanced Performance: Optimize read and write operations for high-volume transactions.
- Scalability: Effortlessly scale your application with NoSQL's distributed architecture.
Sample Code Snippet Link to this section #
Here's how you can convert a simple Java object to a JSON document for MongoDB:
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.HashMap;
import java.util.Map;
public class JavaToNoSQLExample {
public static void main(String[] args) {
ObjectMapper objectMapper = new ObjectMapper();
Map<String, Object> javaObject = new HashMap<>();
javaObject.put("name", "John Doe");
javaObject.put("email", "[email protected]");
try {
String json = objectMapper.writeValueAsString(javaObject);
System.out.println(json); // Output: {"name":"John Doe","email":"[email protected]"}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Resources Link to this section #
Using this tool, you can efficiently transition from traditional relational databases to modern NoSQL systems, aligning with the demands of today's data-intensive applications.
Frequently Asked Questions
How can I connect a Java application to a NoSQL database?
To connect a Java application to a NoSQL database, you typically use a specific NoSQL database driver or client library. For example, if you're using MongoDB, you can use the MongoDB Java Driver. These libraries provide the necessary methods to establish a connection, perform CRUD operations, and handle data within the NoSQL database.
What are the benefits of using NoSQL databases with Java applications?
NoSQL databases offer several benefits when used with Java applications, including scalability, flexibility in data modeling, and the ability to handle large volumes of unstructured data. They are particularly useful for applications that require real-time analytics, distributed data, and horizontal scaling. Java's extensive library support and mature ecosystem make it well-suited for integrating with NoSQL databases.
What are some popular NoSQL databases that can be used with Java?
Some popular NoSQL databases that can be used with Java include MongoDB, Cassandra, Redis, Couchbase, and Amazon DynamoDB. Each of these databases has its own set of features and use cases, ranging from document-based storage in MongoDB to key-value storage in Redis. They all offer Java client libraries to facilitate integration with Java applications.