C Sharp to SQL: A Comprehensive Guide
Introduction
C Sharp (C#) is a powerful programming language used for a variety of applications, including database management. SQL (Structured Query Language) is the standard language for managing and manipulating databases. This article will guide you through the process of connecting C# to SQL, optimizing your code for performance, and ensuring your applications run smoothly.
Table of Contents
- Understanding C# and SQL
- Setting Up Your Environment
- Connecting C# to SQL
- Executing SQL Commands in C#
- Handling SQL Data in C#
- Best Practices for C# to SQL Integration
- Common Errors and Troubleshooting
- FAQs
Understanding C# and SQL
C# is a versatile language developed by Microsoft, widely used for developing Windows applications. SQL, on the other hand, is used to interact with databases. Combining these two allows developers to create robust applications that can store, retrieve, and manipulate data efficiently.
Setting Up Your Environment
Before you start, ensure you have the following:
- Visual Studio installed
- SQL Server installed
- Necessary libraries and packages
Connecting C# to SQL
To connect C# to SQL, you need to use the
System.Data.SqlClient
namespace. Here’s a simple example:
using System;
using System.Data.SqlClient;
class Program
{
static void Main()
{
string connectionString = "your_connection_string";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
Console.WriteLine("Connection Opened");
}
}
}
Executing SQL Commands in C
Once connected, you can execute SQL commands using the
SqlCommand
class. Here’s an example of inserting data:
string query = "INSERT INTO Students (Name, Age) VALUES (@Name, @Age)";
using (SqlCommand command = new SqlCommand(query, connection))
{
command.Parameters.AddWithValue("@Name", "John Doe");
command.Parameters.AddWithValue("@Age", 25);
command.ExecuteNonQuery();
}
Handling SQL Data in C
To retrieve data, use the
SqlDataReader
class:
string query = "SELECT * FROM Students";
using (SqlCommand command = new SqlCommand(query, connection))
{
using (SqlDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
Console.WriteLine($"{reader["Name"]}, {reader["Age"]}");
}
}
}
Best Practices for C# to SQL Integration
- Use Parameterized Queries: Prevent SQL injection by using parameterized queries.
- Connection Management: Always close connections to avoid memory leaks.
- Error Handling: Implement robust error handling to manage exceptions.
Common Errors and Troubleshooting
- Connection Errors: Ensure your connection string is correct.
- SQL Syntax Errors: Double-check your SQL commands for syntax errors.
- Data Type Mismatches: Ensure data types in your C# code match those in your SQL database.
FAQs
How do I connect C# to SQL Server?
Use the
SqlConnection
class from the
System.Data.SqlClient
namespace and provide a valid connection string.
What is a parameterized query in C#?
A parameterized query uses parameters to pass values to SQL commands, preventing SQL injection attacks.
How can I handle SQL exceptions in C#?
Use try-catch blocks to catch and handle SqlException
and other exceptions.
What is the best way to manage database connections in C#?
Use the using
statement to ensure connections are properly closed and disposed of.
Can I use LINQ with SQL in C#?
Yes, LINQ to SQL allows you to use LINQ queries to interact with SQL databases.
Conclusion
Connecting C# to SQL is a fundamental skill for developers working with databases. By following best practices and understanding common pitfalls, you can create efficient and secure applications. Remember to use parameterized queries, manage your connections wisely, and handle errors gracefully.
External Links
- Microsoft Documentation on SqlConnection
- Tutorial on Parameterized Queries
- Best Practices for C# and SQL
By following this guide, you can ensure your C# applications interact seamlessly with SQL databases, providing a robust and efficient solution for data management.
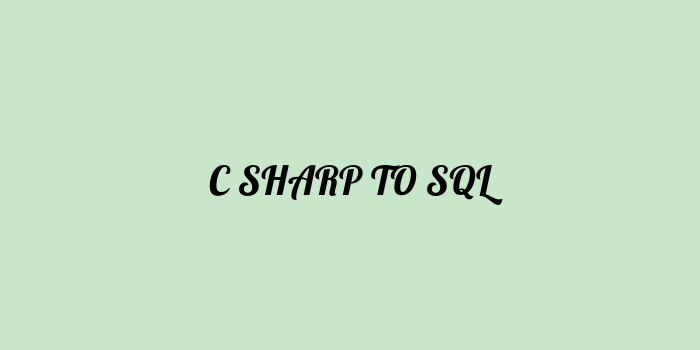