Master Ruby Code Debugging with Our Advanced Tool
Effortlessly debug Ruby code with our advanced Ruby Code Debugger tool. Enhance performance, identify bugs, and streamline your coding process efficiently.
Code to Debug
Debug Results
Output will appear here...
The Ruby Code Debugger is an essential tool for developers, allowing seamless identification and resolution of code errors, enhancing productivity and code quality. With features like breakpoint management and real-time variable inspection, it simplifies the debugging process for Ruby applications. Ideal for both novice and experienced developers, this tool supports efficient error tracking and optimization in Ruby projects.
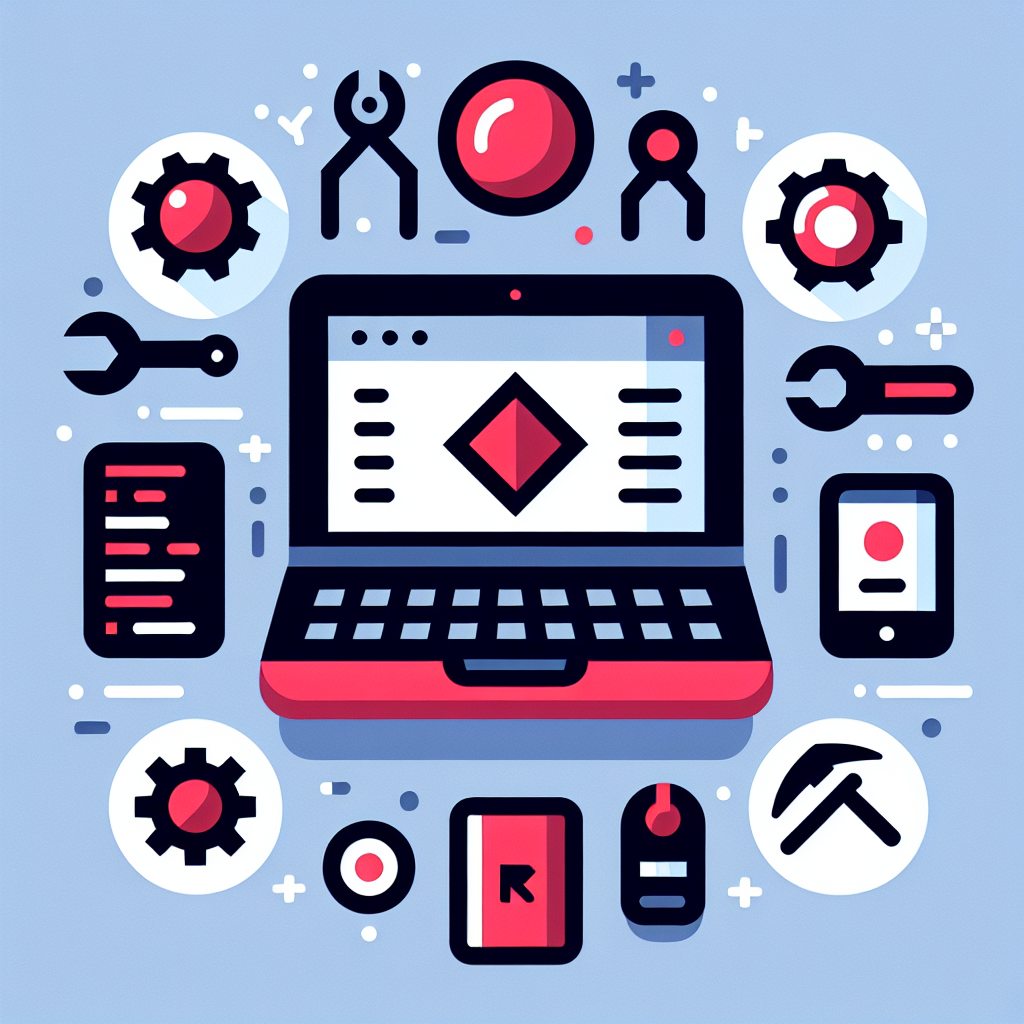
Ruby Code Debugger: A Comprehensive Tool for Developers Link to this section #
The Ruby Code Debugger is an essential tool for software developers seeking to efficiently troubleshoot and refine Ruby programs. This powerful debugger enables you to inspect and manipulate code execution, ensuring your applications run seamlessly. Below are key features and tips for optimizing your debugging process.
Key Features Link to this section #
- Breakpoints: Easily set breakpoints to pause code execution, allowing for detailed inspection of variable states and program flow.
- Step Execution: Navigate through your code with step-by-step execution, enabling precise identification of logic errors.
- Variable Inspection: Access current variable values and modify them on-the-fly to test different scenarios and outcomes.
- Call Stack Navigation: Traverse the call stack to understand the sequence of method calls leading up to a particular point in your program.
Basic Usage Link to this section #
To initiate debugging in a Ruby script, include the following snippet:
require 'byebug'
def example_method
byebug
puts "Hello, World!"
end
example_method
Run your script using a Ruby interpreter. The execution will pause at byebug
, allowing you to inspect the environment with commands like next
, step
, and continue
.
Additional Tips Link to this section #
- Watch Expressions: Monitor specific expressions for changes in value to quickly identify unexpected behavior.
- Logging: Utilize logging for a persistent record of variable states and execution paths Ruby Logging Documentation.
- Interactive Console: Use IRB (Interactive Ruby) in conjunction with the debugger to test code snippets on-the-fly.
Resources Link to this section #
Enhance your debugging skills with these resources:
With these strategies and tools, the Ruby Code Debugger will significantly streamline your development workflow, ensuring efficient and effective debugging for your Ruby applications.
Frequently Asked Questions
What is a Ruby code debugger?
A Ruby code debugger is a tool that helps developers identify and fix bugs in their Ruby applications by allowing them to inspect, control, and manage the execution of their code. It provides features such as breakpoints, step execution, and variable inspection to facilitate the debugging process.
How do I use the Ruby debugger in a Rails application?
To use the Ruby debugger in a Rails application, you can insert a `byebug` statement in your code where you want the execution to pause. Run your Rails server or script, and the execution will stop at the `byebug` line, allowing you to inspect variables, step through code, and evaluate expressions in the console.
What are some popular Ruby debugging tools?
Some popular Ruby debugging tools include Byebug, Pry, and the built-in `debug` library. Byebug provides a simple interface for debugging, Pry offers an interactive REPL with powerful features, and the `debug` library comes with Ruby's standard library for basic debugging tasks.